How to Connect to Cassandra Using Java and JDBC
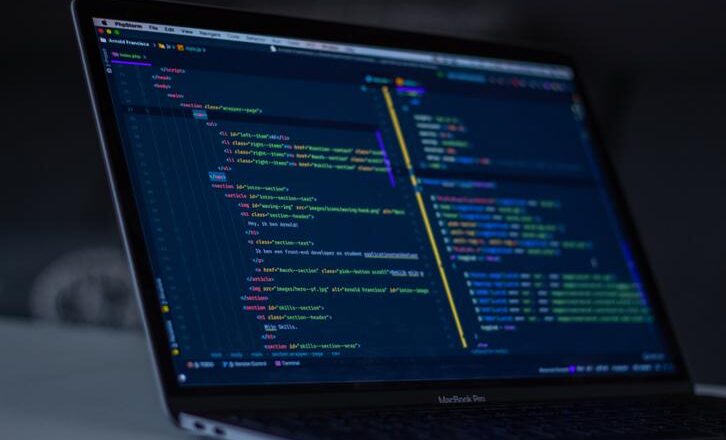
In this tutorial, we will see how to connect to Cassandra using Java and jdbc. Assuming you have a Cassandra instance up and running, we will proceed to an example.
Example – Connect To Cassandra Database Using JDBC
In this example, we will be using Maven to manage the Cassandra dependencies. This article on how to create a Maven project will help if you are not familiar with Maven. You may also skip the maven portion and download the libraries if you prefer. Now, let us list down the high-level steps we are going to do in this example.
- Create a maven project.
- Add the required dependencies to pom.xml.
- Use the CqlSession to create a Cassandra session.
- Execute a simple query using the newly created session.
- Loop through the result sets using Java for each loop.
These are the dependencies we need to add to pom.xml.
<dependency>
<groupId>com.datastax.oss</groupId>
<artifactId>java-driver-query-builder</artifactId>
<version>4.8.0</version>
</dependency>
<dependency>
<groupId>com.datastax.oss</groupId>
<artifactId>java-driver-mapper-processor</artifactId>
<version>4.8.0</version>
</dependency>
<dependency>
<groupId>com.datastax.oss</groupId>
<artifactId>java-driver-mapper-runtime</artifactId>
<version>4.8.0</version>
</dependency>
<dependency>
<groupId>com.datastax.oss</groupId>
<artifactId>java-driver-core</artifactId>
<version>4.8.0</version>
</dependency>
After the maven project creation and adding the dependencies, create a java class “CassandraExample.java”. Now, copy the below code and change the database details to match with your cassandra instance.
import java.net.InetSocketAddress;
import com.datastax.oss.driver.api.core.CqlSession;
import com.datastax.oss.driver.api.core.cql.ResultSet;
import com.datastax.oss.driver.api.core.cql.Row;
public class CassandraExample {
private static String contactPoint = "mycassandradb.hotname.com";
private static int port = 9042;
private static String keySpace = "mydb";
private static String dataCenter = "datacenter1";
public static void main(String args[]) {
try (CqlSession session = CqlSession.builder().addContactPoint(new InetSocketAddress(contactPoint, port))
.withLocalDatacenter(dataCenter).withKeyspace(keySpace).build()) {
ResultSet rs = session.execute("select *from mytable limit 5");
for (Row row : rs.all()) {
System.out.println(row.getString("column_name"));
}
} catch (Exception e) {
System.out.println(e.getMessage());
}
}
}
Since we are using the Java try with resources block, we don’t have to specifically close the database session. Also you will have to change the project compliance settings to a minimum of Java 1.8 in your IDE (eg: Eclipse).